1 method 实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <div id="root"> <h3>今天天气很{{ info }}</h3> <button @click="changeWeather">切换天气</button> </div>
<script type="text/javascript"> Vue.config.productionTip = false; const vm = new Vue({ el:'#root', data:{ isHot:true, }, computed:{ info(){ return this.isHot ? '炎热' : '凉爽' } }, methods: { changeWeather(){ this.isHot = !this.isHot } } }) </script>
|
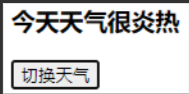
2 watch 实现
监视属性 watch:
- 当被监视的属性变化时, 回调函数自动调用, 进行相关操作
- 监视的属性必须存在,才能进行监视,既可以监视
data
,也可以监视计算属性
- 配置项属性
immediate:false
,改为 true,则初始化时调用一次 handler(newValue,oldValue)
- 监视的两种写法:
new Vue
时传入 watch: {}
配置
- 通过
vm.$watch()
监视
第一种写法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| <div id="root"> <h2>今天天气很{{ info }}</h2> <button @click="changeWeather">切换天气</button> </div>
<script> const vm = new Vue({ el:'#root', data:{ isHot:true, }, computed:{ info(){ return this.isHot ? '炎热' : '凉爽' } }, methods: { changeWeather(){ this.isHot = !this.isHot } }, watch:{ isHot:{ immediate: true, handler(newValue, oldValue){ console.log('isHot被修改了',newValue,oldValue) } } } }) </script>
|
第二种写法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <div id="root"> <h2>今天天气很{{ info }}</h2> <button @click="changeWeather">切换天气</button> </div>
<script> const vm = new Vue({ el:'#root', data:{ isHot:true, }, computed:{ info(){ return this.isHot ? '炎热' : '凉爽' } }, methods: { changeWeather(){ this.isHot = !this.isHot } } });
vm.$watch('isHot',{ immediate:true, handler(newValue,oldValue){ console.log('isHot被修改了',newValue,oldValue) } }) </script>
|
3 深度侦听
- Vue 中的 watch 默认不监测对象内部值的改变(一层)
如 obj:{name:’ds’,age:18}
这里的一层指的后面整个对象字面量,而不是里面的值是第一层
- 配置
deep:true
可以监测对象内部值改变(多层)
备注:
- Vue 自身可以监测对象内部值的改变,但 Vue 提供的 watch 默认不可以
- 使用 watch 时根据数据的具体结构,决定是否采用深度监视
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| <title>天气案例_深度监视</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"> <h3>a的值是:{{ numbers.a }}</h3> <button @click="numbers.a++">点我让a+1</button> <h3>b的值是:{{ numbers.b }}</h3> <button @click="numbers.b++">点我让b+1</button> <button @click="numbers = {a:666,b:888}">彻底替换掉numbers</button> {{numbers.c.d.e}} </div>
<script type="text/javascript"> Vue.config.productionTip = false; const vm = new Vue({ el: '#root', data: { isHot: true, numbers: { a: 1, b: 1, c: { d: { e: 100 } } } }, watch: { handler(){ console.log('a被改变了') } } */ numbers: { deep: true, handler() { console.log('numbers改变了') } } } }) </script>
|
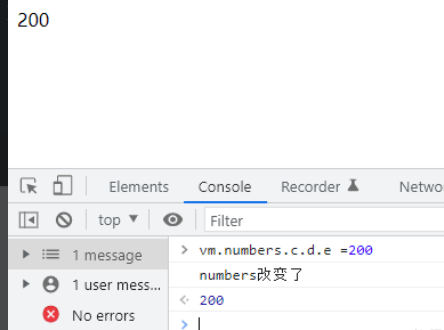
4简写
如果监视属性除了 handler
没有其他配置项的话,可以进行简写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <div id="root"> <h3>今天天气很{{ info }}</h3> <button @click="changeWeather">切换天气</button> </div>
<script type="text/javascript"> Vue.config.productionTip = false; const vm = new Vue({ el: '#root', data: {isHot: true,}, computed: {info() {return this.isHot ? '炎热' : '凉爽'}}, methods: {changeWeather() {this.isHot = !this.isHot}}, watch: { isHot(newValue, oldValue) { console.log('isHot被修改了', newValue, oldValue) } } })
</script>
|
监视到 isHot
改变会自动调用 handler
方法
5 computed 和 watch 之间的区别
- computed 能完成的功能,watch 都可以完成
- watch 能完成的功能,computed 不一定能完成,例如:watch 可以进行异步操作
两个重要的小原则:
- 所被 Vue 管理的函数,最好写成普通函数,这样 this 的指向才是 vm 或 组件实例对象
- 所有不被 Vue 所管理的函数(定时器的回调函数、ajax 的回调函数等、Promise 的回调函数),最好写成箭头函数,这样 this 的指向才是 vm 或 组件实例对象
比如想延迟一秒显示fullName
,只能用watch
实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| new Vue({ el:'#root', data:{ firstName:'张', lastName:'三', fullName:'张-三' }, watch:{ firstName(val){ setTimeout(()=>{ this.fullName = val + '-' + this.lastName },1000); }, lastName(val){ this.fullName = this.firstName + '-' + val } } })
|