1 问题演示
先来个案例引入一下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| <div id="root"> <h2>人员列表</h2> <button @click="updateMei">更新马冬梅的信息</button> <ul> <li v-for="(p,index) of persons" :key="p.id"> {{p.name}}-{{p.age}}-{{p.sex}} </li> </ul> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
const vm = new Vue({ el:'#root', data:{ persons:[ {id:'001',name:'马冬梅',age:30,sex:'女'}, {id:'002',name:'周冬雨',age:31,sex:'女'}, {id:'003',name:'周杰伦',age:18,sex:'男'}, {id:'004',name:'温兆伦',age:19,sex:'男'} ] }, methods: { updateMei(){ this.persons[0] = {id:'001',name:'马老师',age:50,sex:'男'} } } }) </script>
|
点击更新马冬梅的信息,马冬梅的数据并没有发生改变。
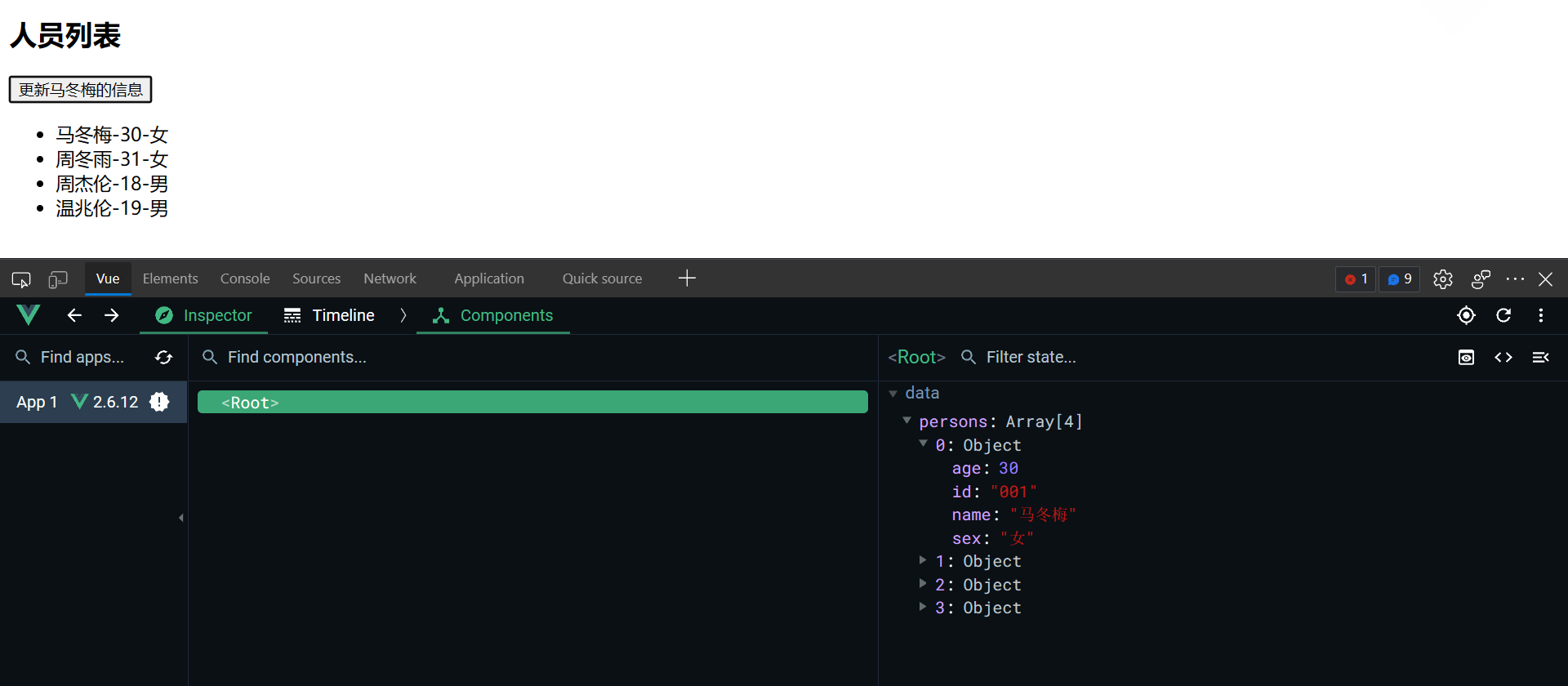
我们来看看控制台:
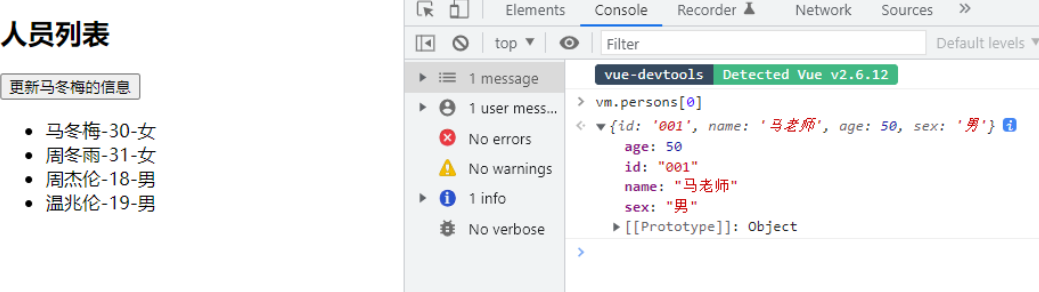
控制台上的数据发生了改变,说明,这个更改的数据并没有被 vue 监测到。
2 模拟一个数据监测
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| let data = { name: '尚硅谷', address: '北京', };
function Observer(obj) { const keys = Object.keys(obj); keys.forEach((k) => { Object.defineProperty(this, k, { get() { return obj[k] }, set(val) { console.log(`${k}被改了,我要去解析模板,生成虚拟DOM.....我要开始忙了`); obj[k] = val } }) }) }
const obs = new Observer(data); console.log(obs);
let vm = {}; vm._data = data = obs
|
3 Vue.set 的使用
Vue.set(target,propertyName/index,value)
或 vm.$set(target,propertyName/index,value)
用法:向响应式对象中添加一个 property,并确保这个新 property 同样是响应式的,且触发视图更新。它必须用于向响应式对象上添加新 property,因为 Vue 无法探测普通的新增 property (比如 vm.myObject.newProperty = 'hi'
)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <div id="root"> <h1>学生信息</h1> <button @click="addSex">添加性别属性,默认值:男</button> <br/> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
const vm = new Vue({ el:'#root', data:{ student:{ name:'tom', age:18, hobby:['抽烟','喝酒','烫头'], friends:[ {name:'jerry',age:35}, {name:'tony',age:36} ] } }, methods: { addSex(){ this.$set(this.student,'sex','男') } } }) </script>
|
Vue.set()
或 vm.$set
有缺陷:对象不能是 Vue 实例。或者 Vue 实例的根数据对象。
4监测数组
看完了 vue 监测对象中的数据,再来看看 vue 如何监测数组里的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <div id="root"> <h2>爱好</h2> <ul> <li v-for="(h,index) in student.hobby" :key="index"> {{h}} </li> </ul> <h2>朋友们</h2> <ul> <li v-for="(f,index) in student.friends" :key="index"> {{f.name}}--{{f.age}} </li> </ul> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
const vm = new Vue({ el:'#root', data:{ student:{ name:'tom', age:{ rAge:40, sAge:29, }, hobby:['抽烟','喝酒','烫头'], friends:[ {name:'jerry',age:35}, {name:'tony',age:36} ] } } methods: {} }) </script>
|
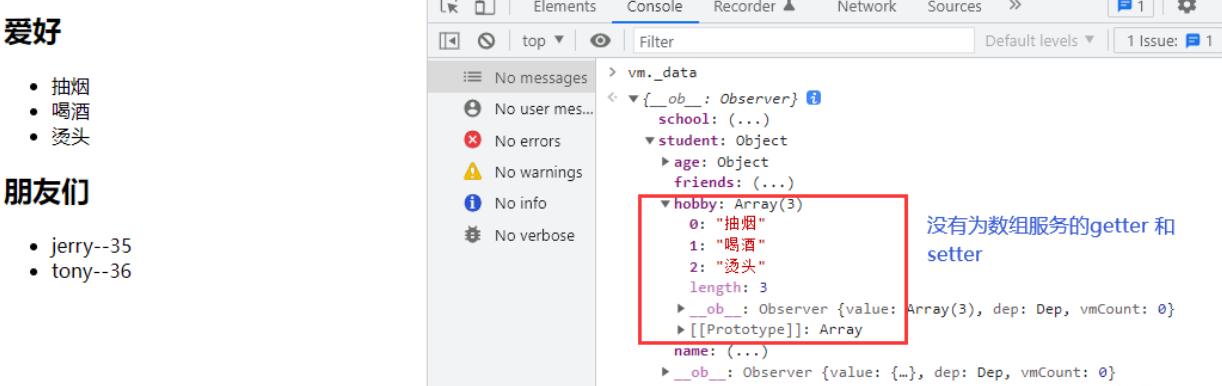
所以我们通过 vm._data.student.hobby[0] = 'aaa' // 不奏效
vue
监测在数组那没有 getter 和 setter,所以监测不到数据的更改,也不会引起页面的更新

既然 vue 在对数组无法通过 getter 和 setter 进行数据监视,那 vue 到底如何监视数组数据的变化呢?
vue 对数组的监测是通过包装数组上常用的用于修改数组的方法来实现的。
vue 官网的解释:https://cn.vuejs.org/guide/essentials/list.html#array-change-detection
5练习
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| <style>button {margin-top: 10px;}</style>
<div id="root"> <h1>学生信息</h1> <button @click="student.age++">年龄+1岁</button> <br /> <button @click="addSex">添加性别属性,默认值:男</button> <br /> <button @click="student.sex = '未知' ">修改性别</button> <br /> <button @click="addFriend">在列表首位添加一个朋友</button> <br /> <button @click="updateFirstFriendName">修改第一个朋友的名字为:张三</button> <br /> <button @click="addHobby">添加一个爱好</button> <br /> <button @click="updateHobby">修改第一个爱好为:开车</button> <br /> <button @click="removeSmoke">过滤掉爱好中的抽烟</button> <br /> <h3>姓名:{{ student.name }}</h3> <h3>年龄:{{ student.age }}</h3> <h3 v-if="student.sex">性别:{{student.sex}}</h3> <h3>爱好:</h3> <ul> <li v-for="(h,index) in student.hobby" :key="index">{{ h }} </li> </ul> <h3>朋友们:</h3> <ul> <li v-for="(f,index) in student.friends" :key="index">{{ f.name }}--{{ f.age }}</li> </ul> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
const vm = new Vue({ el: '#root', data: { student: { name: 'tom', age: 18, hobby: ['抽烟', '喝酒', '烫头'], friends: [ { name: 'jerry', age: 35 }, { name: 'tony', age: 36 } ] } }, methods: { addSex() { this.$set(this.student, 'sex', '男') }, addFriend() { this.student.friends.unshift({ name: 'jack', age: 70 }) }, updateFirstFriendName() { this.student.friends[0].name = '张三' }, addHobby() { this.student.hobby.push('学习') }, updateHobby() { this.$set(this.student.hobby, 0, '开车') }, removeSmoke() { this.student.hobby = this.student.hobby.filter((h) => { return h !== '抽烟' }) } } });
|
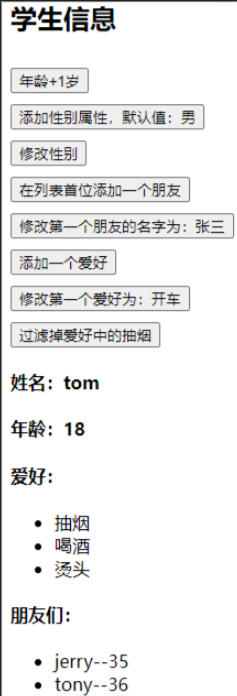
6 总结
Vue监视数据的原理:
vue会监视data中所有层次的数据
如何监测对象中的数据?
通过 setter 实现监视,且要在 new Vue
时就传入要监测的数据。
- 对象中后追加的属性,Vue默认不做响应式处理
- 如需给后添加的属性做响应式,请使用如下API:
Vue.set(target,propertyName/index,value)
或 vm.$set(target,propertyName/index,value)
如何监测数组中的数据?
通过包裹数组更新元素的方法实现,本质就是做了两件事:
- 调用原生对应的方法对数组进行更新
- 重新解析模板,进而更新页面
在 Vue 修改数组中的某个元素一定要用如下方法:
- 使用这些API:push()、pop()、shift()、unshift()、splice()、sort()、reverse()
Vue.set()
或 vm.$set()
特别注意:Vue.set()
和 vm.$set()
不能给 vm 或 vm 的根数据对象添加属性!!!例:Vue.set(this,…,…)
或this.$set(this,…,…)