1 ref属性
ref
被用来给元素或子组件注册引用信息(id的替代者)
- 应用在
html
标签上获取的是真实DOM元素
,应用在组件标签上是组件实例对象vc
- 使用方式:
- 打标识:
<h1 ref="xxx">.....</h1>
或 <School ref="xxx"></School>
- 获取:
this.$refs.xxx
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <template> <div> <h1 v-text="msg" ref="title"></h1> <button ref="btn" @click="showDOM">点我输出上方的DOM元素</button> <School ref="sch"/> </div> </template>
<script> import School from './components/School'
export default { name:'App', components:{ School }, data() { return { msg:'欢迎学习Vue!' } }, methods: { showDOM(){ console.log(this.$refs.title) console.log(this.$refs.btn) console.log(this.$refs.sch) } }, } </script>
|
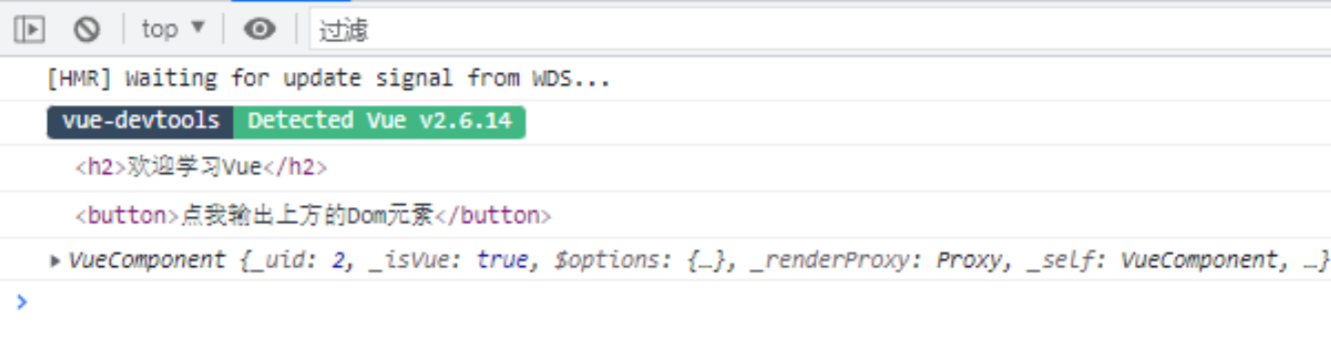
2 props 配置项
功能:让组件接收外部传过来的数据
传递数据:<Demo name="xxx" :age="18"/>
这里age前加:,通过v-bind使得里面的18是数字
接收数据:
- 第一种方式(只接收):
props:['name']
- 第二种方式(限制类型):
props:{name:String, age:Number}
- 第三种方式(限制类型、限制必要性、指定默认值)
1 2 3 4 5 6 7
| props:{ name:{ type:String, //类型 required:true, //必要性 default:'老王' //默认值 } }
|
备注:props是只读的,Vue底层会监测你对props的修改,如果进行了修改,就会发出警告,若业务需求确实需要修改,那么请复制props的内容到data中一份,然后去修改data中的数据。
vue props 传 Array/Object 类型值,子组件报错解决办法
其实看错误信息也就知道了,就是Props在传值类型为Object/Array时,**如果需要配置default
值(如果没有配置default
值,则不会有这个报错)**,那必须要使用函数来return
这个default
值,而不能像基本数据类型那样直接写default:xxx
1 2 3 4 5 6 7
| //错误写法 props: { rlist: { type:Array, default: [1, 2, 3, 4, 5] } }
|
解决方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| //正确写法 props: { rlist: { type:Array, default: function() { return [1, 2, 3, 4, 5] } } } //当然,我们可以使用箭头函数来写,还显得简单很多 props: { rlist: { type:Array, default: () => [1, 2, 3, 4, 5] } }
|
示例代码: 父组件给子组件传数据
App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <template> <div> <Student name="李四" sex="女" :age="18"/> <Student name="王五" sex="男" :age="18"/> </div> </template>
<script> import Student from './components/Student'
export default { name:'App', components:{ Student } } </script>
|
School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| <template> <div> <h1>{{ msg }}</h1> <h2>学生姓名:{{ name }}</h2> <h2>学生性别:{{ sex }}</h2> <h2>学生年龄:{{ myAge + 1 }}</h2> <button @click="updateAge">尝试修改收到的年龄</button> </div> </template>
<script> export default { name: "Student", data() { console.log(this); return { msg: "我是一个bilibili大学的学生", myAge: this.age, }; }, methods: { updateAge() { this.myAge++; }, }, // 简单声明接收 // props:['name','age','sex']
// 接收的同时对数据进行类型限制 // props: { // name: String, // age: Number, // sex: String, // }
// 接收的同时对数据:进行类型限制+默认值的指定+必要性的限制 props: { name: { type: String, //name的类型是字符串 required: true, //name是必要的 }, age: { type: Number, default: 99, //默认值 }, sex: { type: String, required: true, }, }, }; </script>
|
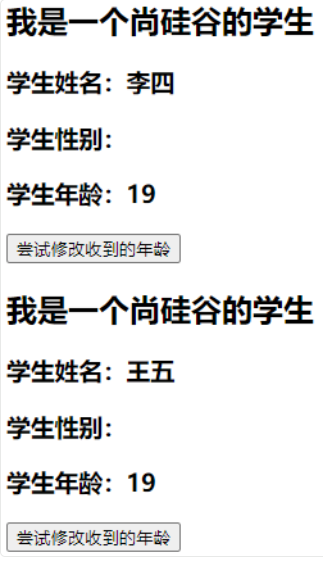
3 mixin 混入
混入 (mixin)
提供了一种非常灵活的方式,来分发 Vue 组件中的可复用功能。一个混入对象可以包含任意组件选项。当组件使用混入对象时,所有混入对象的选项将被“混合”进入该组件本身的选项。
功能:可以把多个组件共用的配置提取成一个混入对象
例子:
1 2 3 4 5
| const mixin = { data() {....}, methods: {....} .... }
|
使用混入
- 全局混入
Vue.mixin(xxx)
- 局部混入
mixins:['xxx']
局部混入
src/mixin.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| export const hunhe = { methods: { showName(){ alert(this.name) } }, mounted() { console.log('你好啊!') }, }
export const hunhe2 = { data() { return { x:100, y:200 } }, }
|
src/components/School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <template> <div> <h2 @click="showName">学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> </div> </template>
<script> //引入一个hunhe import {hunhe,hunhe2} from '../mixin'
export default { name:'School', data() { return { name:'尚硅谷', address:'北京', x:666 } }, mixins:[hunhe,hunhe2] // 局部混入 } </script>
|
src/components/Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <template> <div> <h2 @click="showName">学生姓名:{{name}}</h2> <h2>学生性别:{{sex}}</h2> </div> </template>
<script> import {hunhe,hunhe2} from '../mixin'
export default { name:'Student', data() { return { name:'张三', sex:'男' } }, mixins:[hunhe,hunhe2] // 局部混入 } </script>
|
全局混入
src/main.js
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue' import App from './App.vue' import {mixin} from './mixin'
Vue.config.productionTip = false Vue.mixin(hunhe) Vue.mixin(hunhe2)
new Vue({ el:"#app", render: h => h(App) })
|
这样所有组件就自动使用这些配置了,不推荐使用全局混入
备注
- 组件和混入对象含有同名选项时,这些选项将以恰当的方式进行“合并”,在发生冲突时以组件优先
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| var mixin = { data: function () { return { message: 'hello', foo: 'abc' } } }
new Vue({ mixins: [mixin], data () { return { message: 'goodbye', bar: 'def' } }, created () { console.log(this.$data) } })
|
- 同名生命周期钩子将合并为一个数组,因此都将被调用。另外,混入对象的钩子将在组件自身钩子之前调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| var mixin = { created () { console.log('混入对象的钩子被调用') } }
new Vue({ mixins: [mixin], created () { console.log('组件钩子被调用') } })
|
4 plugin 插件
- 功能:用于增强 Vue
- 本质:包含 install 方法的一个对象,install 的第一个参数是Vue构造函数,第二个以后的参数是插件使用者传递的数据
- 定义插件(见下
src/plugin.js
)
- 使用插件:
Vue.use()
Vue.use
执行之后,会自动调用install
方法,所有组件就可以使用里面定义的东西。
src/plugin.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| export default { install(Vue,x,y,z){ console.log(x,y,z) Vue.directive('fbind',{ bind(element,binding){element.value = binding.value}, inserted(element,binding){element.focus()}, update(element,binding){element.value = binding.value} })
Vue.mixin({ data() {return {x:100,y:200}}, })
Vue.prototype.hello = ()=>{alert('你好啊')} } }
|
src/main.js
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue' import App from './App.vue' import plugins from './plugins'
Vue.config.productionTip = false
Vue.use(plugins,1,2,3)
new Vue({ el:'#app', render: h => h(App) })
|
src/components/School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <template> <div> <h2>学校名称:{{ name | mySlice }}</h2> <h2>学校地址:{{ address }}</h2> <button @click="test">点我测试一个hello方法</button> </div> </template>
<script> export default { name:'School', data() { return { name:'尚硅谷atguigu', address:'北京', } }, methods: { test(){ this.hello() } }, } </script>
|
src/components/Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <template> <div> <h2>学生姓名:{{ name }}</h2> <h2>学生性别:{{ sex }}</h2> <input type="text" v-fbind:value="name"> </div> </template>
<script> export default { name:'Student', data() { return { name:'张三', sex:'男' } }, } </script>
|
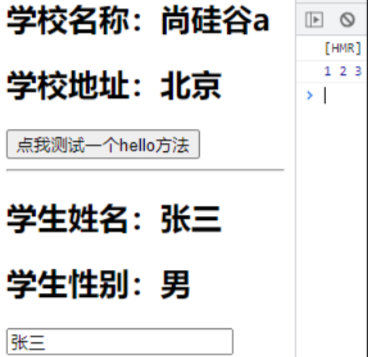
5 scoped
scoped样式
- 作用:让样式在局部生效,防止冲突。
- 写法:
<style scoped>
具体案例:
1 2 3 4 5 6 7 8
| <style lang="less" scoped> .demo{ background-color: pink; .atguigu{ font-size: 40px; } } </style>
|